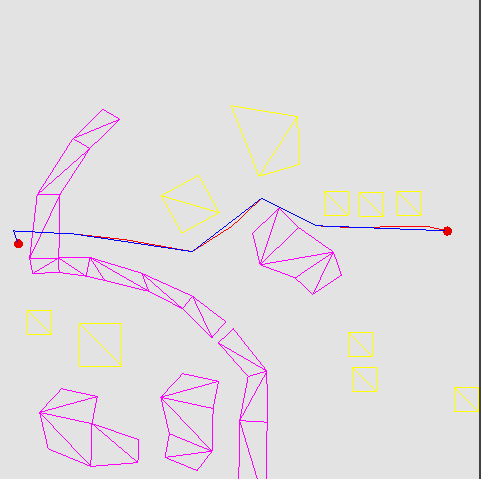
Didn’t do anything yesterday.
Today I added some code to cope with rotated and scaled obstacles (before you had to count on the user having applied scale and rotation to all the obstacles before they ran the game, a recipe for false bugs) I also split the obstacles in to obstructions(yellow) and hindrances(magenta).
Obstructions are buildings and rocks and very thick clumps of trees. No agent, not matter how adept can move through them.
Hindrances are things that hinder your movement. Sticky mud, sparse trees, shallow water, etc… If an agent has the “all_terrain” property it can move through the terrain but at a reduced speed. other agents will get stuck in such terrain so they can’t enter.
This allows things such as infantry trying to swim a cross a stream, or move through a forest. It also allows difficult winter or desert terrain. The A* routine will still try to find a way around such hindrances, preferring a bridge if it is close to the crossing point, or trying to take a path through a forest. On the other hand, if you feel the need you can over ride this behavior by manually ordering the agent to go through the terrain, which will avoid the A* check. For example if the bridge is mined, or there are infantry ready to mount an ambush in the trees.
Finally I modularized the code for the A* initiation and moved it over to my bgeutils.py script so from the main movement script you only have to run:
import bgeutils
bgeutils.navigation_initiate(own)
and it will create the required properties on your AI control handling object. This is part of my drive to clean up my projects, moving things in to a back area where they can do their thing without me having to scroll through them to get to the next big function. Right now I only have the single utility script, but I may use different scripts for different functions, such as AI, Movement, Effects etc… However, there comes a time when scrolling through a couple of hundred lines of code is no more confusing than switching back and forth between 20+ different scripts, some of which overlap in function.
I hope to finish the movement scripts this week, testing them out on a fully 3d map with buildings and difficult terrain.
It’s my goal with this project to concentrate on game design and mechanics and leave the art assets until later as much as I can. Once I have a working game I can relax and start working on visual side.
Today I was thinking back over my past attempts at AI for Blender. One of the first I designed was this one:
It was nearly all made with logic bricks, with about 15% done with python (mostly stolen from other people’s game demos). This was in 2010, when I first started coding. Looking at it now, it’s not that bad. Functional and surprisingly fast for a system that relied on rays and radars. Sure the agents often crash in to each other or in to buildings, but it’s not bad considering. 